React Typescript Cheat Sheet Pdf
- The cheat sheet was created by Kenneth Setzer and released especially for Smashing Magazine and its readers. The cheat sheet is a mousepad-sized image featuring a typical PC keyboard. Photoshop’s keyboard shortcuts are listed for each key, with the shortcut and its icon printed on the individual key.
- All React + TypeScript Cheatsheets The Basic Cheatsheet (/README.md) is focused on helping React devs just start using TS in React apps Focus on opinionated best practices, copy+pastable examples. Explains some basic TS types usage and setup along the way.
- Start your development with a Dashboard for Bootstrap 4, React and Reactstrap, made with create-react-app. It is open source, free and it features many components that can help you create amazing websites.
- The one-page guide to TypeScript: usage, examples, links, snippets, and more. This is Devhints.io cheatsheets — a collection of cheatsheets I've written. TypeScript is just like ES2015 with type-checking. All ES2015 (classes, etc) should work.
Cheatsheet for React Hooks. A cheatsheet with live editable examples 😎. A one-stop reference for hooks 💪.
· 6 mins readTypescript provides some interfaces to help with writing React components. React.FC
is one of those interfaces that is used in a lot of apps to define a functional component. The question you should ask yourself is: should you use React.FC
for Typescript react components?
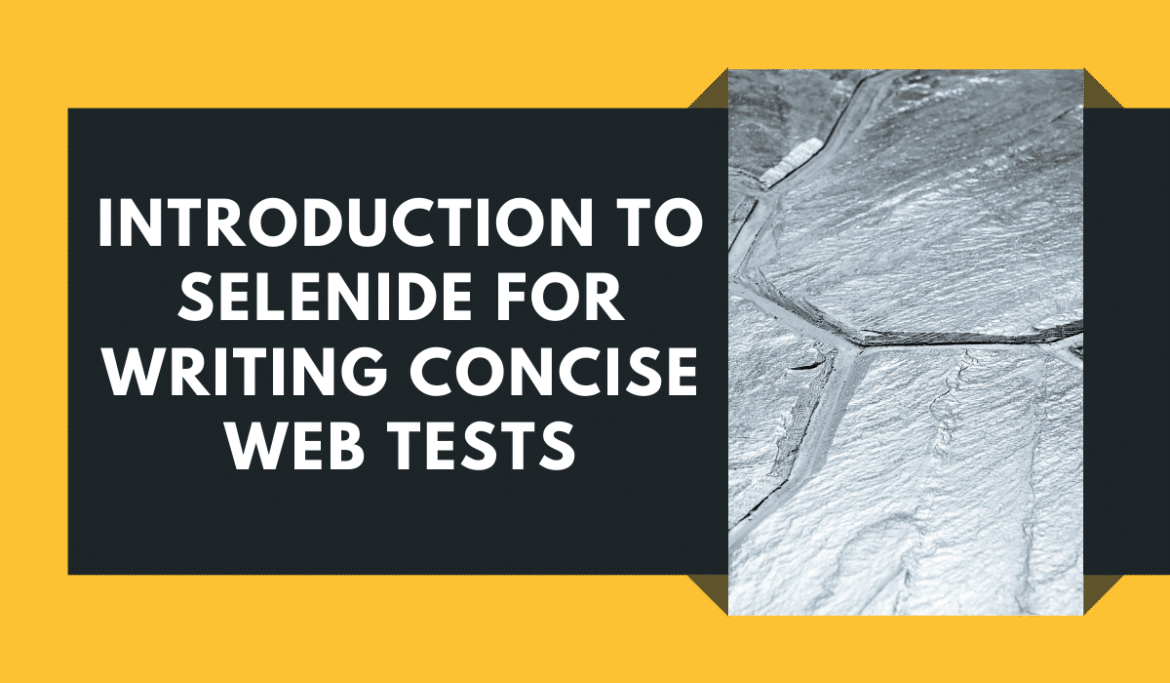
React.FC
This example shows how you can use React.FC
in practice:
and without React.FC
:
- wondering what the
T
is about?
With React component props, use:
React Fc
without
Pros of React.FC
1. Helps with Component props
React components have by default 4 props, e.g. Header.displayName
.
With React.FC
interface I get typings for these props, otherwise we get this type error:
2. Gives access to typed children
without
to fix this, we need to specify the children
prop manually Reddit download lightroom adobe mac.
Cons of React.FC
1. Doesn’t help with returning undefined
You cannot return undefined
from a React component, and even with the React.FC
type, the compiler will only tell you at runtime.
2. Provides access to props.children
when you may not want it
With React.FC
I can put children under my component and they won’t render but will be typed correctly.
Without this type I get a error instantly
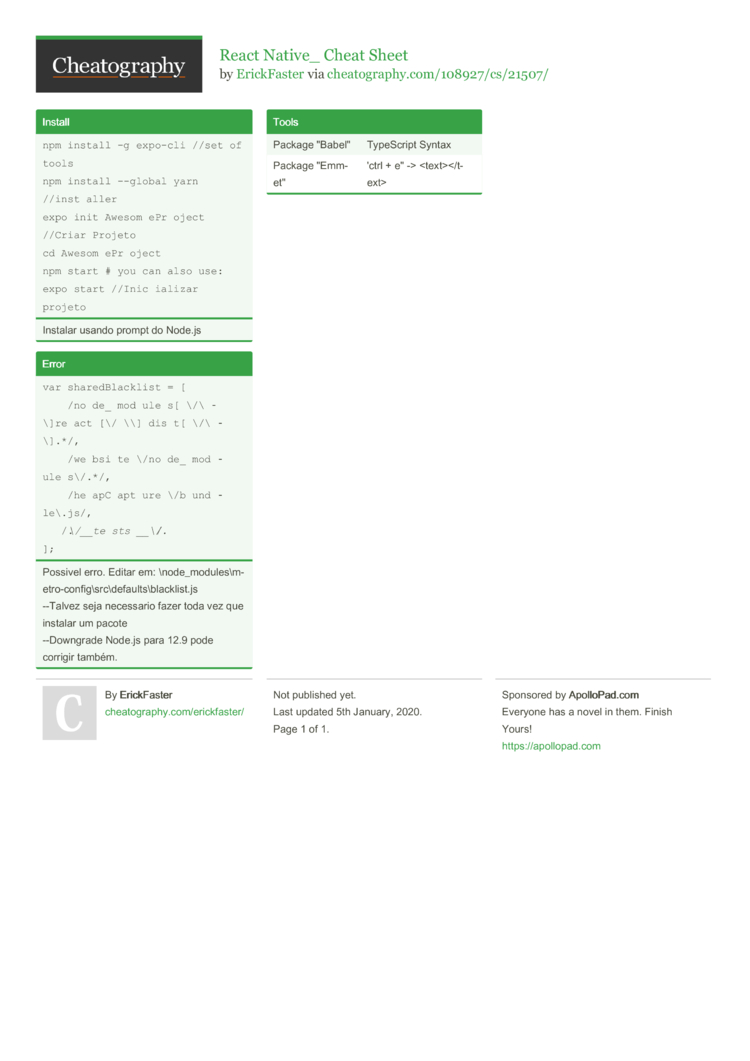
3. Difficulty creating a Sub.Component pattern
React Typescript Cheat Sheet Example
It is very difficult to create the typical Component and Component.SubComponent pattern (without adding lots of additional workarounds) with React.FC
.
e.g. without React.FC
, to create this:
the types are simply
Overall
React.FC
is useful for beginners getting into typed React components as it guides you with types. But due to unnecessary addition of children, that you normally do not need, you should stay away and simply type like a normal Typescript function.
More reading
Suggested
- 7 Best Practices for Cypress — 17 April 2020
I recently refactored and improved a large test suite library that used Cypress as the main test automation tool. Here are some best practices and common mistakes I found for working with this library.
- Learn Frontend in 2021 — 21 December 2020
Frontend engineer changes every year. Different technologies, libraries and techniques. This series is everything you need to know to get up to scratch with Frontend Engineering in 2021.
Written by Harry Mumford-Turner // See the blog code on GitHub
